Published on : 4th Oct 2021
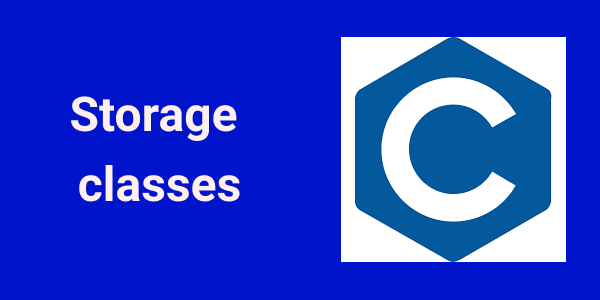
Storage classes in C programming
A variable in C programming belongs to a particlular storage class.
Storage classes tell us :
- Where the variable will be stroed.
- What is the initial value of the variable.
- What is the scope of the variable.
- What is the life of the variable.
There are four storage classes in C programming.
- auto
- register
- static
- extern
Let's discuss them one by one.
1. auto:
Storage : Memory [stack section]
Initial value : Garbage value (an unpredictable value)
Scope : Local to the block where the variable is declared.
Life : Within the block.
Source code :
#include <stdio.h> int main(int argc, char** argv) { auto int num = 100; { printf("Number = %d", num); } return 0; }
Output :
Number = 100
Note : If we don't specify any storage class within the function, it is auto by default.
2. register:
Storage : Register
Initial value : Garbage value (an unpredictable value)
Scope : Local to the block where the variable is declared.
Life : Within the block.
Source code :
#include <stdio.h> int main(int argc, char** argv) { register int i; printf("Numbers = "); for(i=0; i < 5; i++) { printf(" %d", i); if(i != 4) { printf(","); } } return 0; }
Output :
Numbers = 0, 1, 2, 3, 4
Note : Register access is faster than memory access. We cannot access the address of a register variable. So, we cannot use pointer on a register variable.
3. static:
Storage : Memory [static/global section]
Initial value : Zero
Scope : Local to the block where the variable is declared.
Life : Till the end of a program.
Source code :
#include <stdio.h> void func() { static int i; printf("Value = %d\n", i); i++; } int main(int argc, char** argv) { register int i; for(i=0; i<5; i++) func(); return 0; }
Output :
Value = 0 Value = 1 Value = 2 Value = 3 Value = 4
Note : Here, i static variable persists between different function calls.
4. extern:
Storage : Memory [static/global section]
Initial value : Zero
Scope : Global.
Life : Till the end of a program.
Source code :
#include <stdio.h> int i; void func() { extern int i; printf("Value = %d\n", i); i++; } int main(int argc, char** argv) { register int i; for(i=0; i<5; i++) func(); return 0; }
Output :
Value = 0 Value = 1 Value = 2 Value = 3 Value = 4
Note : Here, i extern variable persists until the program ends.