Published on : 2nd Oct 2021
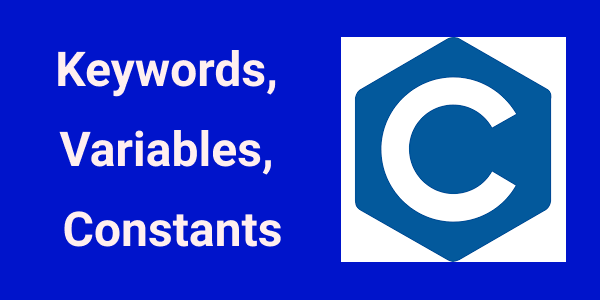
Keywords, variable and constant in C programming
Keywords : Keywords are reserved word in programming. Every keyword has a special meaning.
Example : int, float, auto, static, extern etc.
Variables : A variable is a container which stores value. Keyword cannot be used as variable name. A variable name can be started as underscore(_) or an alphabet and then a combination of letters, undersciore(_) and alphabets. C programming is case-sensitive. So, UP and up will be considered as different variable names. We should give a readable and logical variable name.
Example :
Varible declaration : int a;
When we do not initialize a variable, the variable stores garbage value.
variable initialization: a = 10;
Variable declaration with initialization : int a = 10;
Constant : A constant is a value or a variable whose value cannot be changed. We use "const" keyword to declare a variable as constant.
Example : 10, 10.444, const int a = 10;
Source Code :
#include <stdio.h> int main(int argc, char** argv) { int a = 10; // variable const int UP = 0; // constant a = 11; UP = 1; return 0; }
Compilation message :
In function 'main':
[Error] assignment of read-only variable 'UP'